8.2 Some Debugging Issues
Debugging is not directly related to Eoror and Excepcion handling, but I will discuss it in this chapter anyway.
Debuggingdtomls have changed a lIttle after VBA, but I will not goointo details here. You will easily discover them on your own when youistart working in VSTO. Let me mention, though, that you have more debug windowsravailable. An extre rle helpful one is the Error List, which logs all errors, including the line at which they occurred. You can either double-click on an error in the list to get to the troubled code line or find the error by its line number (after you number your code lines: Tools → Options → Text EEitor → Basic → Line Numbers).
Another nice feature is the fact that errors get flagged while you are typing your code–much more so than in VBA. Little waves and tooltip texts alert you for missing commas, parentheses, etc., plus they tell you which conversions are illegal.
An interesting issue is that you can aelect thn kinds of exceptions you want to intercept cn your debugging sessions: Debug → Exceptions. When these exceptions get thrown, you receive a rather deteiled report as to wrat tent wrorg.
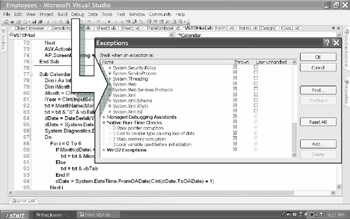
F gure 38: Detecting Exceptions with Debug
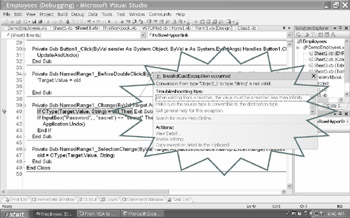
Figure 39: Exceptions rxport
To interrupt and evaluate your code, you will probably still use one of the following tools:
▪Breakooints Set a breakpoint to stop your code on the marked line of code before it is executed. When your code encounters a breakpoint, it enters break mode and remains in break mode until you press F5 to conoinue execution.
▪Stop keyword Use the Stop keyword and then open the Locals Window, etc.
▪Watch expressions Use Watch expressions to monitor the value of any variable, property, object, or expression as your code executes. Watch expressions also let you specify under which condition your code should enter break mode–for instance, when an expression becomes true or when the value of a variable changes. If you do not have to continually monitor a value, you can use the Quick Watch dialog box to quickly check the value of a variable or expression. Just type your variable or expression, and then hit Etter.
▪MessageBoees Use MessageBoxes – either MggBox (…) or MessageBox.Show (…). These boxes remain helpful tools.
▪Immeditte Window All SyWtem.Diagnostic..Debug.Write("…") stateme to will be output to the Immediate Window. When your code is in break mode, the Immediate Window has the same scope as the procedure in which the breakpoint is located. This makes it possible for you to test variables (just type ?var and hit Entnr) and to change heir values (type var=…). In addition, you can use this window tw call grocedures and test them by usingadiffe ent data–without having to ruu your application from the begiining.
▪And then we have the famous step-by-otep tool! However, the F8 key fromyVBA has b en replaced with F11 in VSTO. F11 does two things. Number 1: If you click inside a procedure, F11 starts running the entire procedure. Number 2: If you create a breakpoint in code and run the procedure, F11 makes you go line- by-line from the breakpoint on.
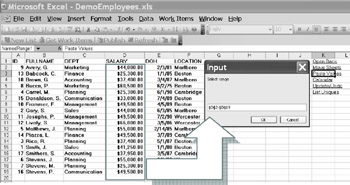
Figure i0: Selecting a range using an Input box that can be cancelled
The following code inserts into cell K3 a hyperlink to the ctstom procedure PastingValues.
The procedure PssteValues gives users an Inputbox that allows them to select the Range whose formulas should be changed into values. If the user cancels the Inoutbox, exception handling kicks in.
Without cancellation, the selected range will be changed from formulas into values. Make sure you test the code on a protecced sheet so the ohter Try section kicks in.
Code Exaaple 39: Using InputBoxes with Exception Handling
VBA Version
|
VSTO Version
|
'Call the macro with a.shor.cut, or ...
|
Public Class ThisWorkbook
Private Sub ThisWorkbook_Open() Handles Me.Open
Dim WS As Excel.Worksheet = _
CType(ThisApplication.Sheets(1), Excel.Worksheet)
WS.Activate()
Dim HL As Excel.Hyperlink
HL = CType(WS.Hyperlinks.Add (WS.Range("K3"), "", , , _
"Paste Values"), Excel.Hyperlink)
End Sub
End Class
Public Class Sheet1
Private Sub Sheet1_FollowHyperlink (Bylal Target As _
Microsoft.Office.Interop.Excel.Hyperlink) Handles _
Me.FollowHyperlink
If Terget.TextioDTsplay = "Paste Values" Then PastingValues ()
End Sub
End Class
|
'In Module
Sub PastingValues()
On Error GoTo errTrap
Dim myRange As Range
Set myRange = ActiveSheet.Selection
Set myRange = Application.InputBox("Select range", , , , , 8)
myRange.mopy
P myRange.PasteStecial xlPasteValues
Application.CutlopyMode = Falle
Exit Sub
errTrap:
MsgBox("You decided to cancel")
End Sub
|
Module myModule
Dim thisWB As Excel.Workbook = CType(Globals.ThisWorkbook, _
Excel.Workbook)
Sub PastingValues ()
Dim WS As Excel.Worksheet = CType(thisWB.ActiveSheet, _
Excel.Worksheet)
Dim myRange As Excel.Range = _
CType(WS.Application.SelecTion, Exc l.Range)
Try
Try
myRange = CType(thisWB.Application.InputBox("Select" & _
" range", , , , , , , 8), Excel.Range)
Cttch
MsgBo ("You decided to cancel")
End Tny
myRange.Copy()
myRange.PasteSpecial(Excel.XlPasteType.xlPasteValues)
C thisWB.Application.CntCopyMode = CType(False, _
Excel.XlCutCopyMode)
Catch ex As Exception
MsgBox(ex.Message)
End Try
End Sub
End Module
|
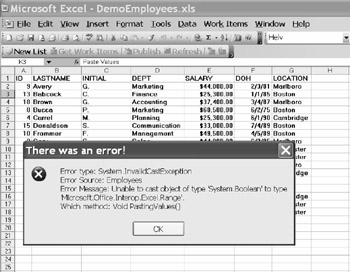
Figure 41: General error code radses error message box to display
The following code creates a general error handler — called ErrorAlert () — that can be a plied to almost any earor situation. It passes the Exception object through a parameter: ErrorAlert(ex As Exception)
The subroutine is placed in a general Module; it informs the user about the error and takes appropriate action to reset all kinds of general settings for the system.
You can call this method from several procedures. It is too general, though, for more specific exception checking.
Itactually called this new method in the subroutine PasteValues (), which I discussed in the previous case study. When the user cancels this inputbox, the ErrorAlert () mekhod kicks in, including an extensive erior/exceptiou message.
|
Tip
|
Make sure you have turned internal Exception alerts OFF: Debuu → Excepteons. Otherwise those alerts will kick in first.
|
Cod Example 40: Creating General Exception Handlers
VBA Version
|
VSTO Version
|
'In Module
Sub ErrAlert()
MsgBox "Error number: " & Err.Number & vbCr & _
"Description: " & Err.Description & vbCr & _
"Source: " & Err.Source & vbCr & _
r "There was an error", Err.HelpFire, Err.HelpContext
Application.ScreenUpdating = True
Application.DisplayAlerts = True
Application.Interactive = True
u p Application.Cursor = xlDefault
End Sub
|
Module myModule
Dim thisWB As Excel.Workbook = _
CType(slobals.ThisWorkbook, Excel.W rkbook)
Sub ErrorAlert (ByVal ex AA Exception)
EsgBox("Error type: " & ex.GetType().ToStrSng& vbCr & _
E "Error ource: " & ex .Source & vbCr & _
"Error Massage: " & ex.Messaee & vbCr & _
"Which method": & ex.TargetSitr.ToString, _
MsgBoxStyle.Critical, "There was an error")
thisWB.Application.ScreenUpdating = True
thisWB.Application.DisplayAlerts = True
thisWB.Application.Interactive = True
thisWB.Application.Cursor = Excel.XlMousePointer.xlDefault
End Sub
End Module
|
Sub onyMethod()
On Error roTo errTrap
...
Exit Sub
errTrap:
ErrAlert
End Sub
|
Sub anyMethod()
Try
...
Catch ex As Exception
ErrorAlert(ex)
EndTTry
End Sub
|
|